MOST COMMON Uber CODING INTERVIEW QUESTIONS
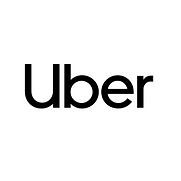
The 15 most asked questions in an uber interview
ARRAYS
Find the missing number in the array
Problem statement
You are given an array containing ‘n’ distinct numbers taken from the range 0 to ‘n’. Since the array has only ‘n’ numbers out of the total ‘n+1’ numbers, find the missing number.
${title}
Determine if the sum of two integers is equal to the given value
Problem statement
Given an array of integers and a value, determine if there are any two integers in the array whose sum is equal to the given value.
LINKED LISTS
Merge two sorted linked lists
Problem statement
Given two sorted linked lists, merge them so that the resulting linked list is also sorted.
${title}
Copy linked list with arbitrary pointer
Problem statement
You are given a linked list where the node has two pointers. The first is the regular ‘next’ pointer. The second pointer is called ‘arbitrary_pointer’ and it can point to any node in the linked list.
Your job is to write code to make a deep copy of the given linked list. Here, deep copy means that any operations on the original list (inserting, modifying and removing) should not affect the copied list.
TREES
Level order traversal of a binary tree
Problem statement
Given the root of a binary tree, display the node values at each level. Node values for all levels should be displayed on separate lines.
${title}
Determine if a binary tree is a binary search tree
Problem statement
Given a Binary Tree, figure out whether it’s a Binary Search Tree. In a binary search tree, each node’s key value is smaller than the key value of all nodes in the right subtree, and are greater than the key values of all nodes in the left subtree i.e. L < N < R.
STRINGS
String segmentation
Problem statement
Given a dictionary of words and an input string tell whether the input string can be completely segmented into dictionary words.
${title}
Reverse words in a sentence
Problem statement
Given a string, find the length of the longest substring which has no repeating characters.
DYNAMIC PROGRAMMING
How many ways can you make change with coins and a total amount
Problem statement
Given coin denominations and total amount, find out the number of ways to make the change.
MATH AND STATS
Find Kth permutation
Problem Statement
Given a set of ‘N’ elements, find the Kth permutation.
${title}
Find all subsets of a given set of integers
Problem statement
You are given a set of integers and you have to find all the possible subsets of this set of integers.
BACKTRACKING
Print balanced brace combinations
Problem statement
Print all braces combinations for a given value 'N' so that they are balanced.
GRAPHS
Clone a directed graph
Problem statement
Given the root node of a directed graph, clone this graph by creating its deep copy so that the cloned graph has the same vertices and edges as the original graph.
SORTING AND SEARCHING
Find the High/Low index
Problem Statement
Given an array of points in the a 2D plane, find ‘K’ closest points to the origin.
${title}
Search rotated array
Problem statement
Given an unsorted array of numbers, find the top ‘K’ frequently occurring numbers in it.