most common ${COMPANY} coding interview questions
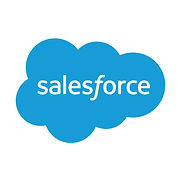
Microsoft Coding Interview Questions
${title}
I'm a paragraph. Click here to add your own text and edit me. It's easy.
I'm a paragraph. Click here to add your own text and edit me. It's easy.
${title}
I'm a paragraph. Click here to add your own text and edit me. It's easy.
I'm a paragraph. Click here to add your own text and edit me. It's easy.
${title}
I'm a paragraph. Click here to add your own text and edit me. It's easy.
I'm a paragraph. Click here to add your own text and edit me. It's easy.
${title}
I'm a paragraph. Click here to add your own text and edit me. It's easy.
I'm a paragraph. Click here to add your own text and edit me. It's easy.
${title}
I'm a paragraph. Click here to add your own text and edit me. It's easy.
I'm a paragraph. Click here to add your own text and edit me. It's easy.
${title}
I'm a paragraph. Click here to add your own text and edit me. It's easy.
I'm a paragraph. Click here to add your own text and edit me. It's easy.
${title}
I'm a paragraph. Click here to add your own text and edit me. It's easy.
I'm a paragraph. Click here to add your own text and edit me. It's easy.
${title}
I'm a paragraph. Click here to add your own text and edit me. It's easy.
I'm a paragraph. Click here to add your own text and edit me. It's easy.
${title}
I'm a paragraph. Click here to add your own text and edit me. It's easy.
I'm a paragraph. Click here to add your own text and edit me. It's easy.
${title}
I'm a paragraph. Click here to add your own text and edit me. It's easy.
I'm a paragraph. Click here to add your own text and edit me. It's easy.
${title}
I'm a paragraph. Click here to add your own text and edit me. It's easy.
I'm a paragraph. Click here to add your own text and edit me. It's easy.
${title}
I'm a paragraph. Click here to add your own text and edit me. It's easy.
I'm a paragraph. Click here to add your own text and edit me. It's easy.