Getting hired at Meta isn’t just about writing code—it’s about solving problems efficiently, designing scalable systems, and demonstrating strong collaboration skills. With a rigorous hiring process, Meta challenges candidates across coding, system design, and behavioral interviews. This guide will break down key interview questions, provide solutions, and share insights to help you prepare effectively.
Meta coding interview questions
The technical screen is the first stage of Meta’s interview process, designed to assess your coding ability and problem-solving skills. The coding interviews consist of algorithmic and data structure-based questions ranging from easy to medium-hard difficulty levels, focusing on efficiency, code clarity, and logical thinking.
Let’s look at some sample questions you might encounter.
1. Two Sum
Problem statement: Given an array of integers, nums
, and an integer target
, return the indexes of any two numbers such that they add up to target
.
Solution: A hash map stores numbers and their indexes while iterating the list.
Code explanation:
- Iterate through the list while storing values and their indexes in a hash map.
- Check if the complement exists in the hash map; return the indexes.
- Otherwise, add the current number to the hash map.
Time complexity: O(n)
Space complexity: O(n)
2. Valid Parentheses
Problem statement: Given a string s
containing only (
,)
, {
, }
, [
and ]
, determine if the input string is valid.
Solution: Use a stack to track open brackets and ensure proper closing.
Code explanation:
- terate through the string, pushing open brackets to a stack.
- If a closing bracket is encountered, check if it matches the last open bracket.
- If mismatched or extra brackets exist, return
False
.
Time complexity: O(n)
Space complexity: O(n)
3. Merge Intervals
Problem statement: Given an array of intervals where intervals[i] = [start, end]
, merge overlapping intervals.
Solution: Sort intervals and merge overlapping ones using a single pass.
Code explanation:
- Sort intervals based on start time.
- Iterate through, merging overlapping intervals.
- If intervals don’t overlap, add them to the result.
Time complexity: O(n log n)
Space complexity: O(n)
More Meta coding interview questions
- Lowest Common Ancestor of a Binary Tree: Given a binary tree, find the lowest common ancestor (LCA) The lowest common ancestor is defined between two nodes p and q as the lowest node in T that has both p and q as descendants (where we allow a node to be a descendant of itself). of two given nodes in the tree.
- Merge k-Sorted Lists: Given an array of
k
linked lists,lists
, each linked list is sorted in ascending order. Your task is to merge all the linked lists into one sorted linked list and return it. - Making a Large Island: You are given an
n x n
binary matrixgrid
and can change at most one 0 to 1. Your task is to return the size of the largest island An island is a 4-directionally connected group of 1s.of in thegrid
after applying this operation. - Valid Palindrome III: Given a string
s
and an integerk
, returntrue
ifs
is ak
-palindrome. A string is ak
-palindrome if it can be transformed into a palindrome by removing at mostk
characters from it. - Maximum Average Subarray I: You are given an integer array
nums
consisting ofn
elements and an integerk
. Find a contiguous subarray whose length equalsk
with the maximum average value and return this value.
System Design interview questions at Meta
System Design interviews at Meta test your ability to design scalable, efficient, and reliable systems. These questions often focus on architecture, trade-offs, high-level problem-solving, and crucial concepts in System Design Interviews.
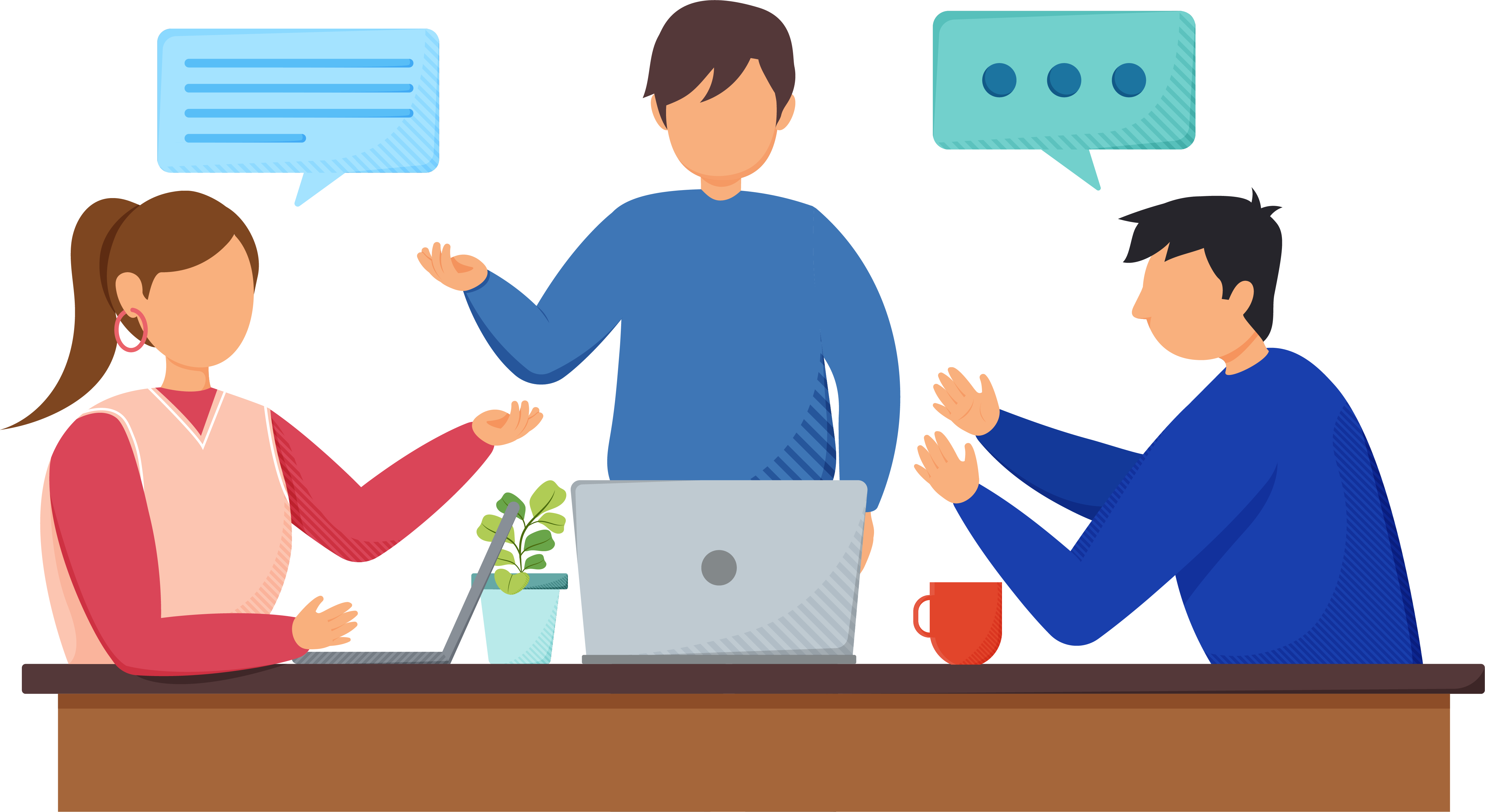
1. Design a rate limiter
Problem: Build a system that limits the number of requests a user can make in a given period.
Key features:
- Prevent abuse by limiting requests based on time windows (e.g., 100 requests per minute).
- Provide immediate feedback on exceeded limits.
- Optional: Different limits for user tiers (e.g., free vs. premium).
Design highlights:
- Use algorithms like token bucket or leaky bucket.
- Store counters in a distributed cache (e.g., Redis).
- Distribute rate-limiting state across multiple servers using a shared cache like Redis.
2. Design a file upload system
Problem: Build a system that handles large file uploads with scalability and fault tolerance.
Key features:
- Support for uploading, storing, and downloading large files.
- Handle multiple concurrent uploads with efficient storage.
- Optional: Add file versioning and metadata.
Design highlights:
- Use object storage (e.g., S3) for scalability.
- Implement file chunking and parallel uploads.
- Ensure fault tolerance by storing redundant copies of files.
3. Design a messaging queue
Problem: Build a scalable and reliable messaging system.
- Publish-subscribe model for real-time communication.
- Message persistence and retries for reliability.
- Handle millions of messages per second.
Design highlights:
- Use Apache Kafka or RabbitMQ.
- Implement message replication for fault tolerance.
- Optimize message delivery with batching.
Additional system design interview questions
- Design a URL shortener: Build a system that shortens URLs and allows efficient redirection.
- Design a video streaming platform: Build a scalable system that delivers high-quality video content to millions of users while ensuring minimal latency.
- Design an E-commerce system: Build a scalable e-commerce platform with search, cart, and checkout functionalities.
- Design a distributed cache: Develop a system to enhance database performance and reduce latency.
- Design a chat application: Develop a scalable system for real-time messaging.
Behavioral interview questions at Meta
Behavioral interview questions at Meta are designed to assess how you handle real-world situations and challenges in the workplace. These questions focus on your ability to collaborate, manage uncertainty, and meet expectations under pressure. A great way to structure your responses is by using the STAR method. The approach helps candidates provide clear, concise, and impactful answers by outlining the context of the challenge (situation), the specific role (task), the steps taken (action), and the outcome (result). Below are some sample questions to help you practice and refine your answers:
1. Handling conflict in a team
Question: Describe a time you had a conflict with a team member and how you resolved it.
STAR answer:
- Situation: I worked on a project where a colleague insisted on using an inefficient approach.
- Task: Needed to collaborate while ensuring the best technical solution.
- Action: Had a one-on-one discussion, presented data on efficiency, and proposed a hybrid solution.
- Result: The team agreed, leading to a 30% performance improvement and stronger collaboration.
2. Managing ambiguity
Question: Tell me about when you had to work on an unclear or ambiguous task.
STAR answer:
- Situation: Assigned a project with unclear requirements in a new domain.
- Task: Needed to define scope and expectations.
- Action: Conducted research, interviewed stakeholders, and proposed an iterative approach.
- Result: Delivered a successful project that aligned with business needs.
3. Handling tight deadlines
Question: Describe a situation where you had to meet a tight deadline.
STAR answer:
- Situation: A last-minute request for an urgent feature implementation.
- Task: Complete the feature while maintaining quality.
- Action: Prioritized tasks, streamlined development, and collaborated closely with QA.
- Result: Delivered on time, preventing business disruption.
Additional behavioral questions to prepare
- Tell me about a time you received critical feedback. How did you handle it?
- Describe a situation where you had to influence a team without direct authority.
- Tell me about a time you made a mistake at work. How did you handle it, and what did you learn?
- Tell me about a time you had to balance multiple priorities. How did you manage them?
- Describe a project where you had to collaborate across different teams. What challenges did you face?
Refine your skills with AI-powered mock interviews
To enhance your preparation, try practicing with an AI-powered mock interview platform like those offered by Educative. These interviews simulate real interview scenarios, offering instant feedback to help you fine-tune your responses to both technical and behavioral questions. With personalized insights, you can refine your performance and build confidence before the actual interview.
What’s next?
Succeeding in Meta’s interviews requires more than just coding practice. You need a strategic approach—mastering fundamental problem-solving techniques, understanding system design principles, and articulating strong behavioral responses. By refining your approach, you can significantly improve your chances of success.
To level up your preparation, consider the following valuable resources:
Company Interview Questions