top of page
most common ${COMPANY} coding interview questions
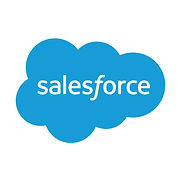
15 Most-Asked Amazon Interview Questions With Answers
Amazon is a technology-driven company that relies on its software infrastructure. The giant e-commerce platform needs experts who can optimize its ecosystem. To land your dream job, you must prove your coding skills during the Amazon interview process. If you need help figuring out where to start the preparation, this guide is the perfect place. To help you, we have compiled a list of the top 15 coding questions asked during Amazon interviews. The answers will teach you the fundamental concepts required to navigate difficult problem-solving questions. They will also help you sharpen your coding abilities so that you can learn to code with confidence during the interview.
bottom of page